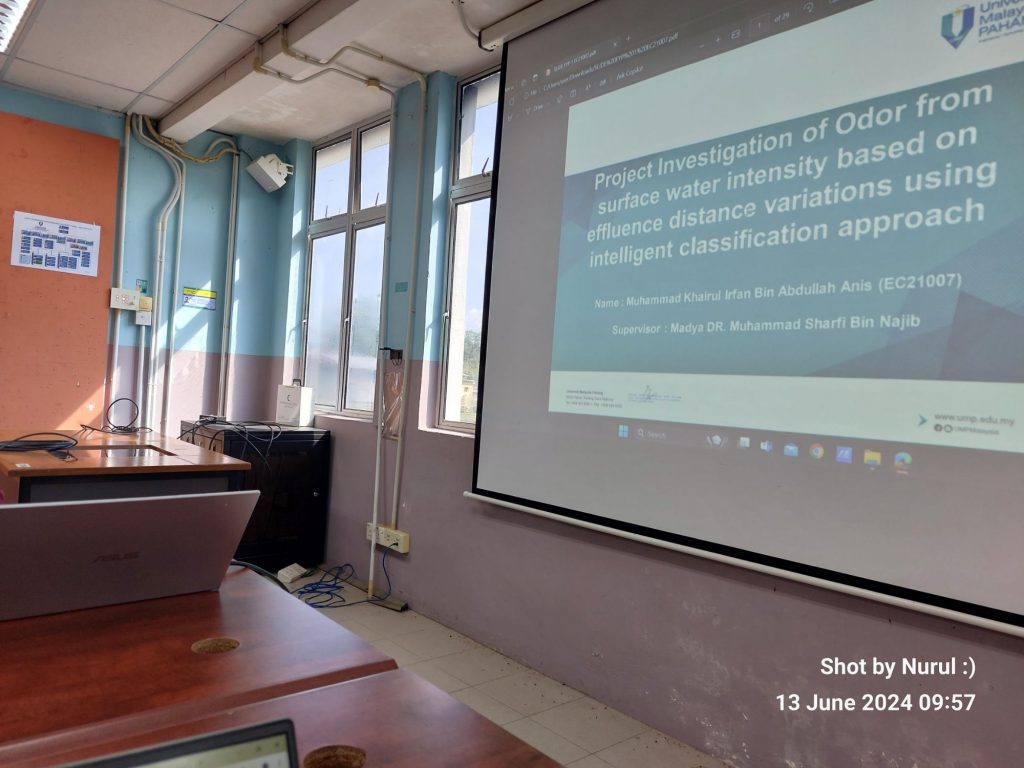
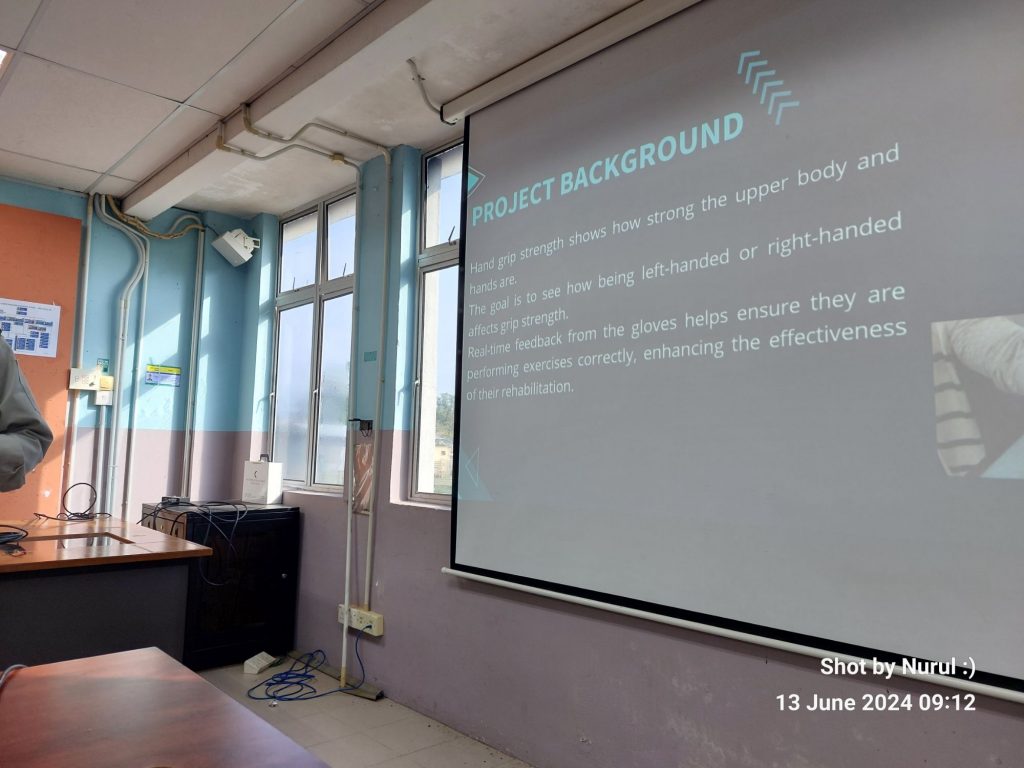
The world is digital, but life is analog..
All the best everyone
Today’s BTE 1522 class was an exciting dive into the world of GPS technology and its integration with Raspberry Pi. We explored the essential concepts, from importing relevant Python modules for GPS functionality to circuit construction and function coding. This session is a crucial part of our project development series, providing students with hands-on experience in incorporating GPS modules into their Raspberry Pi projects.
Global Positioning System (GPS) is a satellite-based navigation system that provides geolocation and time information to a GPS receiver anywhere on or near the Earth. Integrating GPS into Raspberry Pi projects opens up numerous possibilities, such as tracking, navigation, and location-based services, which are pivotal in various applications like autonomous vehicles, drones, and IoT devices.
Components Needed:
Steps:
Connecting the GPS Module:
Powering Up: Power on the Raspberry Pi and ensure all connections are secure.
Python Modules:
gpsd
: A popular library for interfacing with GPS modules.serial
: A library for handling serial communication.UART Communication:
Installing Libraries:
sudo apt-get install gpsd gpsd-clients python-gps
Python Code Example:
import gpsd
# Connect to the local gpsd
gpsd.connect()
# Get GPS data
def get_gps_data():
packet = gpsd.get_current()
latitude = packet.lat
longitude = packet.lon
time = packet.time
return latitude, longitude, time
# Print GPS data
lat, lon,
timestamp = get_gps_data()
print(f"Latitude: {lat}, Longitude: {lon}, Time: {timestamp}")
I2C Communication:
Enabling I2C:
sudo raspi-config
Navigate to ‘Interfacing Options’ and enable I2C.
Python Code Example:
import smbus2
import time
bus = smbus2.SMBus(1)
address = 0x42 # Replace with your GPS module's I2C address
def read_gps():
data = bus.read_i2c_block_data(address, 0, 16)
return data
while True:
gps_data = read_gps()
print(gps_data)
time.sleep(1)
UART (Universal Asynchronous Receiver-Transmitter):
I2C (Inter-Integrated Circuit):
Integrating GPS functionality into Raspberry Pi projects offers a robust foundation for creating advanced IoT applications and location-based services. Today’s class provided a comprehensive understanding of the hardware setup and coding techniques necessary to harness GPS data effectively. As we move forward, these skills will be crucial for the final project development phase, enabling students to implement sophisticated and innovative solutions.